Exploring the Power of Flutter: Building Beautiful Cross-Platform Apps
Outline
1. Introduction to Flutter
2. Setting Up the Development Environment
3. Basics of Flutter App Development
- Installing Flutter and Dart
- Creating Your First Flutter Project
- Understanding Widgets and Flutter Architecture
4. Building User Interfaces with Flutter
- Using Material Design and Cupertino Widgets
- Working with Layouts and Responsive Design
- Adding Interactivity with Gestures and Animations
5. Managing State in Flutter
- Understanding Stateful and Stateless Widgets
- Using setState for Simple State Management
- Advanced State Management with Provider and Riverpod
6. Navigating Between Screens
- Implementing Navigation and Routing in Flutter
- Passing Data Between Screens
- Handling Navigation with Named Routes
7. Working with Data and APIs
- Fetching and Displaying Data from APIs
- Parsing JSON and Working with REST APIs
- Using Packages for Network Requests and Data Persistence
8. Flutter and Firebase Integration
- Setting Up Firebase for Flutter
- Implementing Authentication and User Management
- Using Firebase Services for Storage, Database, and Cloud Functions
9. Testing and Debugging in Flutter
- Writing Unit Tests and Widget Tests
- Using the Flutter DevTools for Debugging and Performance Profiling
10. Deploying and Publishing Flutter Apps
- Preparing the App for Release
- Building and Signing Android and iOS Apps
- Publishing to Google Play Store and Apple App Store
11. Conclusion
12. FAQs
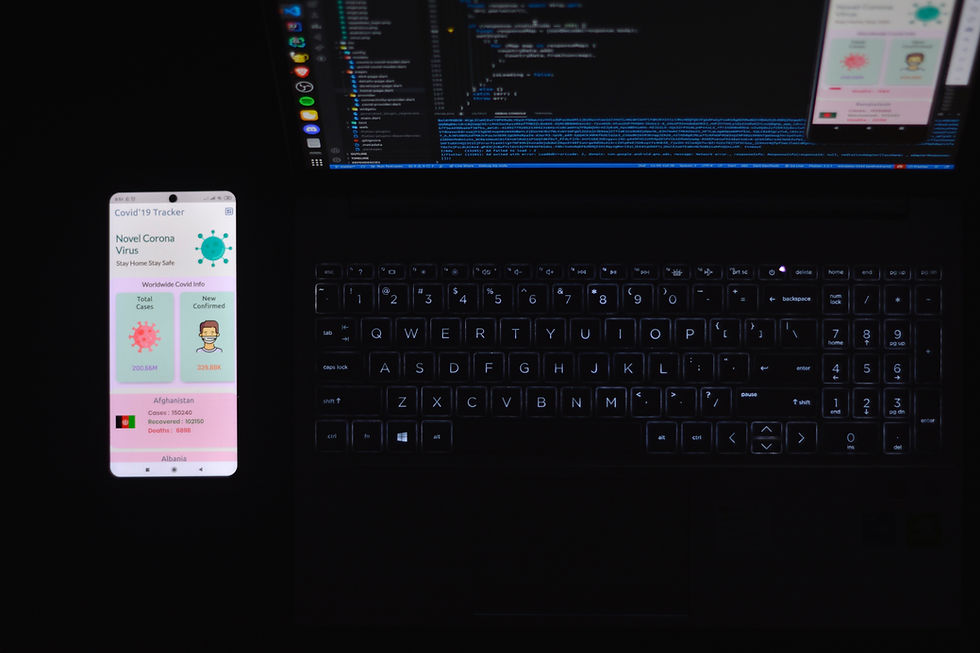
Article
1. Introduction to Flutter
Flutter is an open-source UI software development kit (SDK) created by Google that allows developers to build natively compiled applications for mobile, web, and desktop platforms from a single codebase. It uses the Dart programming language and provides a rich set of pre-designed widgets and tools that enable developers to create beautiful, high-performance apps with a native look and feel.
2. Setting Up the Development Environment
To get started with Flutter app development, you need to set up your development environment. Follow these steps:
Install Flutter and Dart: Download and install Flutter SDK and Dart programming language on your machine.
Configure IDE: Set up an Integrated Development Environment (IDE) like Android Studio or Visual Studio Code with the necessary Flutter and Dart plugins.
Verify Installation: Run a few Flutter commands to ensure the installation is successful.
3. Basics of Flutter App Development
Before diving into advanced topics, it's essential to understand the basics of Flutter app development. Here's what you need to know:
- Installing Flutter and Dart
To begin developing Flutter apps, you need to install Flutter SDK and Dart programming language. Follow the official Flutter documentation for detailed installation instructions.
- Creating Your First Flutter Project
Create a new Flutter project using the command-line tool or IDE. This generates a basic Flutter app structure with sample code that you can modify and build upon.
- Understanding Widgets and Flutter Architecture
Widgets are the building blocks of Flutter apps. Learn about different types of widgets, their properties, and how to compose them to create user interfaces. Understand Flutter's reactive architecture and the concept of a widget tree.
4. Building User Interfaces with Flutter
One of Flutter's strengths is its ability to create beautiful and responsive user interfaces. Explore the following topics:
- Using Material Design and Cupertino Widgets
Flutter provides two sets of widgets: Material Design widgets for Android-style UI and Cupertino widgets for iOS-style UI. Learn how to use these
widgets to create visually appealing and platform-specific UI components.
- Working with Layouts and Responsive Design
Design flexible layouts that adapt to different screen sizes and orientations. Utilize Flutter's layout widgets like Container, Row, Column, Stack, and Flex to arrange UI elements. Implement responsive design principles to ensure your app looks great on various devices.
- Adding Interactivity with Gestures and Animations
Make your app interactive and engaging by incorporating gestures like taps, swipes, and long presses. Use Flutter's animation framework to create smooth and visually pleasing animations that enhance the user experience.
5. Managing State in Flutter
State management is a crucial aspect of building complex apps. Flutter provides different approaches to managing the state based on your app's requirements. Explore the following concepts:
- Understanding Stateful and Stateless Widgets
Understand the difference between stateful and stateless widgets in Flutter. Learn when and how to use each type of widget based on whether the UI element needs to maintain and update its state.
- Using setState for Simple State Management
For simple apps, Flutter's built-in setState method can be used to manage state changes within a widget. Learn how to update widget state and trigger UI updates using setState.
- Advanced State Management with Provider and Riverpod
For larger and more complex apps, consider using state management solutions like Provider and Riverpod. These libraries help manage the state across multiple widgets and provide a scalable and maintainable approach to state management.
6. Navigating Between Screens
Most apps have multiple screens and require navigation between them. Flutter provides various navigation techniques. Explore the following topics:
- Implementing Navigation and Routing in Flutter
Learn how to navigate between screens using Flutter's built-in navigation mechanisms like Navigator.push and Navigator.pop. Understand how to manage the navigation stack and handle screen transitions.
- Passing Data Between Screens
Pass data between screens when navigating to share information and maintain app state. Learn different approaches like constructor arguments, route arguments, and global state management.
- Handling Navigation with Named Routes
Implement navigation using named routes for better organization and maintainability. Define named routes for each screen and navigate using route names instead of directly pushing widgets onto the navigation stack.
7. Working with Data and APIs
Many apps rely on external data sources and APIs to fetch and display data. Learn how to work with data and APIs in Flutter:
- Fetching and Displaying Data from APIs
Use Flutter's http package or other third-party packages to make HTTP requests and fetch data from RESTful APIs. Parse JSON responses and display the retrieved data in your app's UI.
- Parsing JSON and Working with REST APIs
Understand how to parse JSON data received from APIs into Dart objects using Flutter's built-in json package or other JSON parsing libraries. Learn best practices for making API requests and handling errors.
- Using Packages for Network Requests and Data Persistence
Leverage popular packages like Dio, retrofit, or chopper for simplified network requests and API interactions. Explore packages like sqflite or shared_preferences for data persistence and local storage.
8. Flutter and Firebase Integration
Firebase provides a suite of backend services that can be seamlessly integrated into Flutter apps. Learn how to integrate Flutter with Firebase:
- Setting Up Firebase for Flutter
Create a Firebase project and configure it for use with your Flutter app. Set up Firebase Authentication, Firestore or Realtime Database, and other Firebase services as per your app's requirements.
- Implementing Authentication and User Management
Add user authentication to your app using Firebase Authentication. Implement features like email/password login, social login (e.g., Google or Facebook), and user management. Securely store user data and manage user sessions.
- Using Firebase Services for Storage, Database, and Cloud Functions
Explore Firebase services like Cloud Storage, Firestore, Realtime Database, and Cloud Functions. Learn how to upload and retrieve files from storage, store and retrieve data from Firestore/Realtime Database, and use Cloud Functions for serverless backend functionality.
9. Testing and Debugging in Flutter
Ensure the quality and stability of your Flutter app through testing and debugging practices:
- Writing Unit Tests and Widget Tests
Write unit tests to validate the behavior of individual functions or classes. Use widget tests to verify the UI components' correctness and responsiveness. Explore testing frameworks like flutter_test and tools like mockito for effective testing.
- Using the Flutter DevTools for Debugging and Performance Profiling
Learn how to use the Flutter DevTools to debug and profile your app's performance. Analyze app performance, track UI rendering, inspect widget trees, and identify and fix performance bottlenecks.
10. Deploying and Publishing Flutter Apps
When your Flutter app is ready for release, follow these steps to deploy and publish it:
- Preparing the App for Release
Optimize your app for release by removing debug-specific code, enabling release mode, and optimizing assets. Ensure all dependencies and packages are up to date.
- Building and Signing Android and iOS Apps
Generate the APK file for Android or build the iOS app archive (IPA) for iOS. Sign the app using the appropriate certificates and keys. Follow platform-specific guidelines for app signing and release.
- Publishing to Google Play Store and Apple App Store
Upload your app to the Google Play Store for Android users or the Apple App Store for iOS users. Follow the respective app store guidelines, provide necessary app metadata and assets, and submit your app for review.
11. Conclusion
In conclusion, Flutter is a powerful framework for app development that allows you to build high-quality, cross-platform apps using a single codebase. With its rich set of widgets, excellent performance, and robust ecosystem, Flutter provides a smooth development experience. By following the roadmap outlined in this article, beginners can gradually master Flutter and create impressive apps for various platforms.
12. FAQs
Q: Can I use Flutter to build iOS-only or Android-only apps?
A: Yes, Flutter allows you to build apps exclusively for either iOS or Android platforms if needed. However, its true power lies in its ability to create cross-platform apps that run on both platforms from the same codebase.
Q: Is Dart a difficult programming language to learn for Flutter development?
A: Dart is a modern and easy-to-learn programming language. Its syntax is similar to languages like JavaScript and Java, making it relatively straightforward for developers to grasp, especially if they have prior experience with object-oriented programming.
Q: Are there any limitations to using Flutter for app development?
A: While Flutter offers numerous advantages, there are a few limitations to consider. Flutter's ecosystem, although rapidly growing, may have fewer libraries or packages compared to more established frameworks. Additionally, Flutter's performance may vary on older devices or complex animations that require heavy processing.
Q: Can I use Flutter to build web and desktop applications?
A: Yes, Flutter has expanded its capabilities beyond mobile app development. You can use Flutter to build web and desktop applications as well. Flutter for web and Flutter for desktop are in the experimental stage but already provide promising features for multi-platform development.
Q: Is Flutter suitable for building large-scale or enterprise-level apps?
A: Yes, Flutter is suitable for building large-scale or enterprise-level apps. Flutter's modular architecture and state management solutions like Provider and Riverpod make it scalable and maintainable. Many well-known companies have successfully built and deployed large-scale apps using Flutter.
Comments